Tuesday, April 1, 2025
React Native over the air (OTA) updates with custom user interface
Posted by
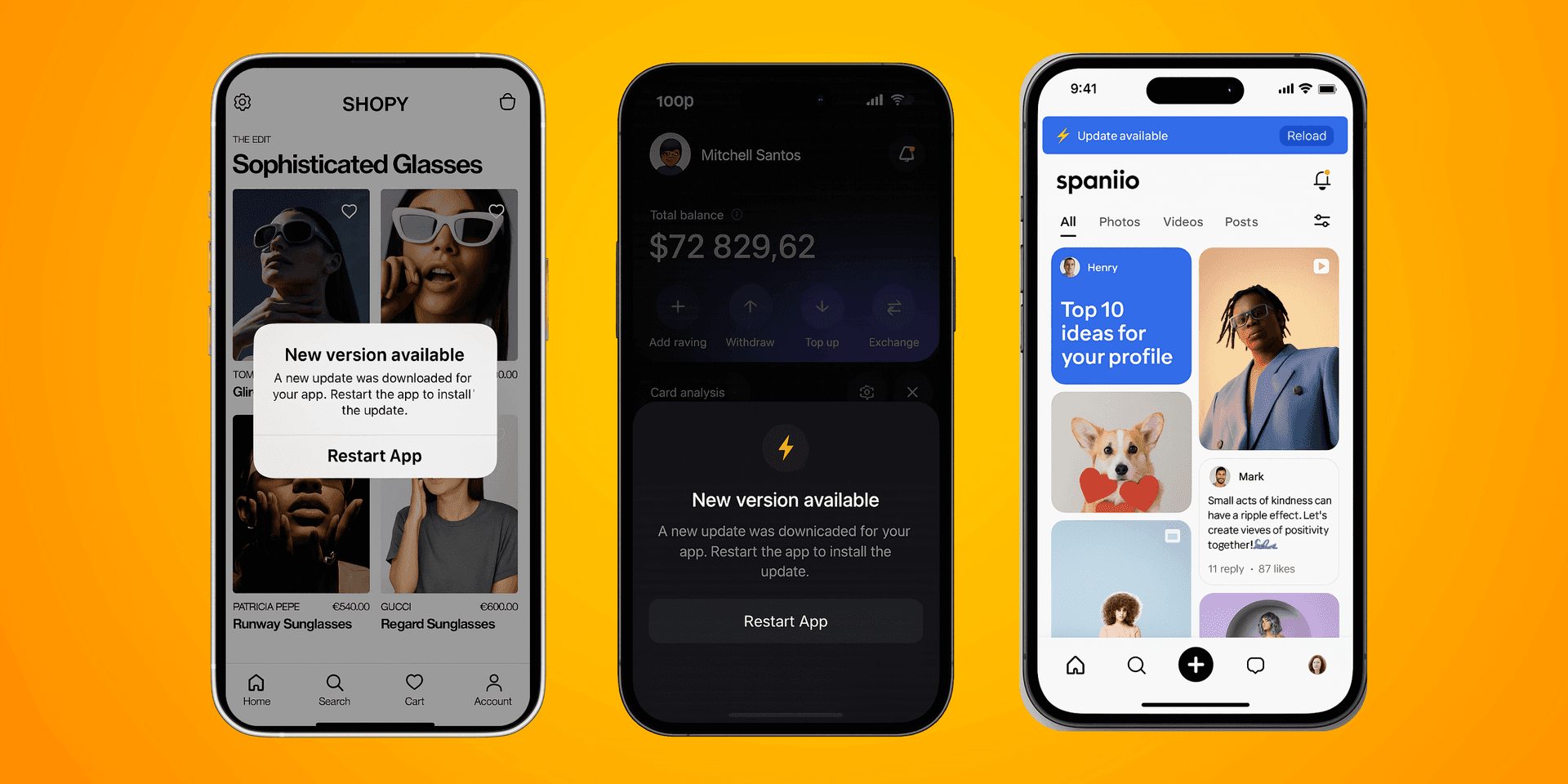
Introduction
Over-the-air (OTA) updates are a core part of modern mobile release strategies for React Native teams. But shipping an update is just half the job—delivering it with the right user experience is what makes the difference.
This blog walks through how to build custom UI prompts—like modals or banners—when a new Stallion OTA update is available. These UI flows can prompt users to restart their app, improving visibility and adoption of your latest releases.
Why Custom UI Matters for OTA
While Stallion can silently fetch and apply updates in the background, you may want to:
- Show a modal when a new update is downloaded
- Display release notes or update version info
- Prompt users to restart the app manually
This kind of control improves transparency, avoids user confusion, and gives you more flexibility.
Detecting OTA Updates with Stallion
Stallion automatically checks for updates when your app moves from background to foreground.
You can also manually check for updates anytime using:
import Stallion from "react-native-stallion";
Stallion.sync(); // Triggers a manual update check
Once an update is downloaded, it’s stored in memory. It will be applied on the next restart.
useStallionUpdate
Triggering Custom UI with You can detect when an update is ready to apply using the useStallionUpdate
hook.
import { useStallionUpdate } from "react-native-stallion";
const MyUpdateChecker = () => {
const { isRestartRequired } = useStallionUpdate();
useEffect(() => {
if (isRestartRequired) {
// Trigger modal or banner here
}
}, [isRestartRequired]);
return null;
};
Minimal Example: Restart Modal
import React, { useEffect, useState } from "react";
import { Modal, Text, View, Button } from "react-native";
import { useStallionUpdate, restart } from "react-native-stallion";
const UpdateModal = () => {
const { isRestartRequired } = useStallionUpdate();
const [modalVisible, setModalVisible] = useState(false);
useEffect(() => {
if (isRestartRequired) {
setModalVisible(true);
}
}, [isRestartRequired]);
const handleRestart = () => {
setModalVisible(false);
restart(); // Trigger Stallion restart
};
return (
<Modal transparent visible={modalVisible}>
<View
style={{
flex: 1,
justifyContent: "center",
alignItems: "center",
backgroundColor: "#00000088",
}}
>
<View
style={{ backgroundColor: "#fff", padding: 20, borderRadius: 10 }}
>
<Text style={{ marginBottom: 10 }}>
A new update is ready to install.
</Text>
<Button title="Restart App" onPress={handleRestart} />
</View>
</View>
</Modal>
);
};
UX Best Practices
- Only show modals for major updates
- Keep messages simple and clear
- Avoid interrupting critical flows
- Use consistent CTAs like “Restart App”
Full working example video
Conclusion
Customizing your OTA update flow ensures better transparency and a smoother experience for your users. Stallion’s JS API makes it easy to tailor how and when updates appear.
📘 Full API Docs: https://learn.stalliontech.io/docs/sdk/api-reference
🚀 Start Here: https://stalliontech.io
Pro Tip:
Let users know when something magical is about to happen—custom UI makes updates more human.